Table of contents
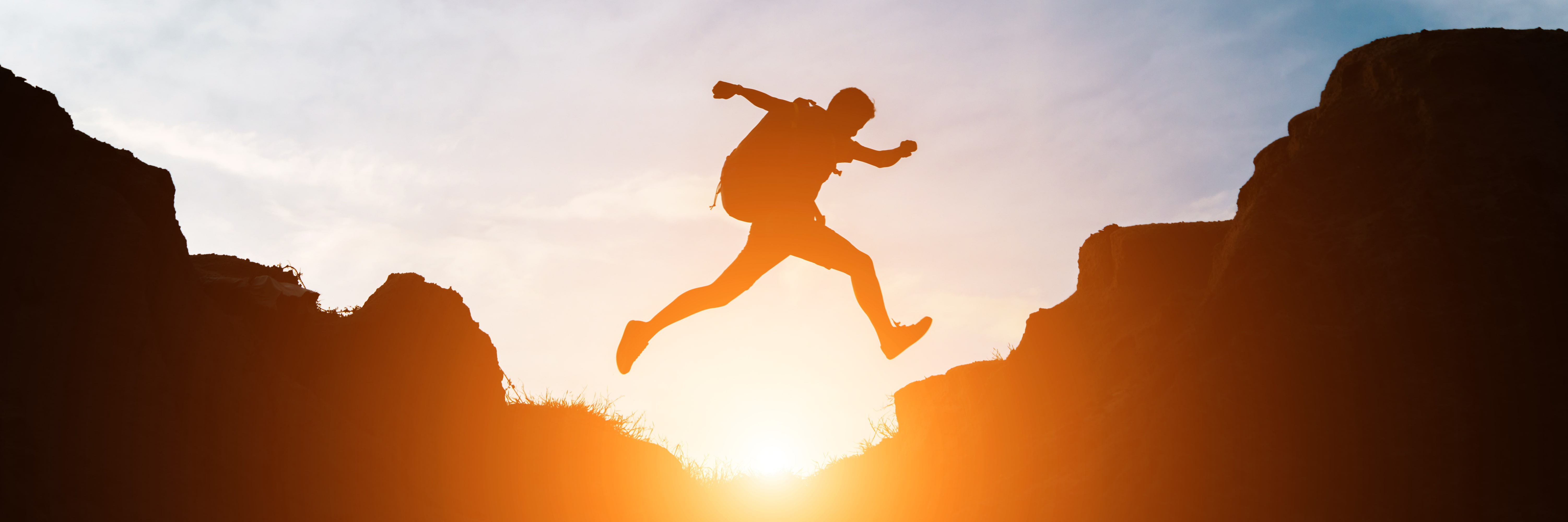
Interpreted vs Compiled Programming Languages
Software Engineer
Software Engineer
Programming languages serve as powerful tools for developers to create software applications and bring their ideas to life. Behind the scenes, these languages are executed by either compiling or interpreting processes. In this blog, we will explore the concepts of compilation and interpretation, shedding light on their differences and how they are applied in popular programming languages like Python, Java, JavaScript, and C++.
Compilation
Compilation is a process where the source code of a program is transformed into machine code, which the computer's hardware can directly execute. It involves several steps:
- Lexical Analysis: The compiler analyzes the source code and breaks it down into smaller meaningful units called tokens. This process is known as lexical analysis or tokenization. Tokens can include keywords, identifiers, operators, constants, etc.
- Syntax Analysis: The compiler then performs syntax analysis, also known as parsing. It checks whether the arrangement of tokens follows the rules defined by the language's grammar. This step ensures that the code is structurally correct and creates a parse tree or an abstract syntax tree (AST).
- Semantic Analysis: In this phase, the compiler examines the semantics or meaning of the code. It checks for any logical errors or inconsistencies that cannot be identified during syntax analysis. The type compatibility, variable declarations, scoping rules, and other language-specific rules are verified at this stage.
- Code Optimization: Once the code has been analyzed and verified, the compiler may perform code optimization. Optimization techniques aim to improve the efficiency of the generated machine code by reducing redundant operations, eliminating unreachable code, or rearranging instructions to enhance performance.
- Code Generation: The final step of compilation involves generating machine code or bytecode. Machine code is specific to the target hardware architecture, whereas bytecode is an intermediate representation that can be interpreted by a virtual machine. The generated code is ready for execution.
Interpretation
Interpretation, unlike compilation, involves executing the source code directly without prior conversion into machine code. Here's how it works:
- Lexical Analysis: Similar to compilation, interpretation starts with lexical analysis, breaking down the source code into tokens.
- Parsing and Execution: Once the tokens are generated, the interpreter reads and analyzes them line by line, executing the code in real-time. It follows the control flow of the program, interpreting each statement and performing the corresponding actions.
- Just-In-Time (JIT) Compilation: Some interpreted languages, like JavaScript, employ a technique called Just-In-Time (JIT) compilation. JIT compilation dynamically translates frequently executed code segments into machine code for improved performance. The interpreter identifies hotspots in the code and optimizes them by converting them into machine code on the fly.
Key Differences
- Execution Speed: Compiled languages tend to execute faster than interpreted languages since the compilation process optimizes the code in advance. Interpreted languages, on the other hand, have the overhead of analyzing and executing the code simultaneously.
- Portability: Interpreted languages, often using bytecode or a virtual machine, offer better portability as they can be executed on any system with a compatible interpreter. Compiled languages generate machine code specific to the target hardware and may require recompilation for different platforms.
- Development Workflow: Compilation provides early detection of syntax and semantic errors before the program runs, helping developers identify issues during the development phase. Interpretation allows for dynamic and interactive programming, enabling developers to experiment and modify code on the go without the need for a separate compilation step.
- Debugging: Interpreted languages generally offer better debugging capabilities, as the interpreter can provide detailed runtime information, such as variable values and stack traces. Compiled languages may require additional debugging tools or techniques to extract similar information.
Example
Interpreted language - JavaScript
JavaScript is primarily an interpreted language. Web browsers have built-in JavaScript engines that interpret and execute JavaScript code. When a web page containing JavaScript is loaded, the browser's engine reads and interprets the code line by line, executing it in real-time. Modern JavaScript engines, like V8 in Chrome, use Just-In-Time (JIT) compilation techniques to optimize performance by dynamically translating frequently executed code segments into machine code.
Compiled language C++
C++ programs follow a traditional compilation process, similar to Java, but with a few differences. C++ source code is compiled by a compiler (such as GCC or Clang) into machine code specific to the target architecture. The resulting binary can then be executed directly on the machine. Unlike Java, C++ does not rely on a virtual machine or interpretation at runtime. The compilation process ensures high performance but sacrifices portability, as the compiled binary is platform-dependent.
Combine both compilation and interpretation
In practice, many modern programming languages and environments combine both compilation and interpretation techniques to strike a balance between performance and flexibility:
JavaScript is primarily an interpreted language. Web browsers have built-in JavaScript engines that interpret and execute JavaScript code. When a web page containing JavaScript is loaded, the browser's engine reads and interprets the code line by line, executing it in real-time. Modern JavaScript engines, like V8 in Chrome, use Just-In-Time (JIT) compilation techniques to optimize performance by dynamically translating frequently executed code segments into machine code.
Compiled language C++
C++ programs follow a traditional compilation process, similar to Java, but with a few differences. C++ source code is compiled by a compiler (such as GCC or Clang) into machine code specific to the target architecture. The resulting binary can then be executed directly on the machine. Unlike Java, C++ does not rely on a virtual machine or interpretation at runtime. The compilation process ensures high performance but sacrifices portability, as the compiled binary is platform-dependent.
Combine both compilation and interpretation
In practice, many modern programming languages and environments combine both compilation and interpretation techniques to strike a balance between performance and flexibility:
- Python, being an interpreted language, does not undergo a traditional compilation process. However, it does undergo a form of compilation known as "bytecode compilation". When a Python script is run, it is first compiled into bytecode, which is a low-level representation of the code. This bytecode is then executed by the Python interpreter. This approach allows Python to be platform-independent, as the bytecode can be executed on any system with a compatible interpreter.
- Java programs are compiled into bytecode using the Java compiler (javac). The resulting bytecode is platform-independent and can be executed on any system with the Java Virtual Machine (JVM) installed. The JVM acts as an interpreter for the bytecode, converting it into machine code at runtime. This process of compiling Java source code into bytecode allows for "write once, run anywhere" portability.
Created at
2023-06-10 11:12:09 UTC
Related blogs
How to Manage and Renew SSL Certificates Using Docker Compose
Securing your website with SSL certificates is essential to protect data transmitted between the server and client. Managing and renewing SSL certific...
Software Engineer
Software Engineer
2024-05-26 08:44:03 UTC
Solving the "must appear in the GROUP BY clause" Error in Rails and PostgreSQL
This blog will give an example of how to resolve this common error when using group_by in Active Record
1. Example problemFirst, take a look at this S...
Software Engineer
Software Engineer
2024-07-10 04:17:29 UTC