Table of contents
Reading Settings
16px
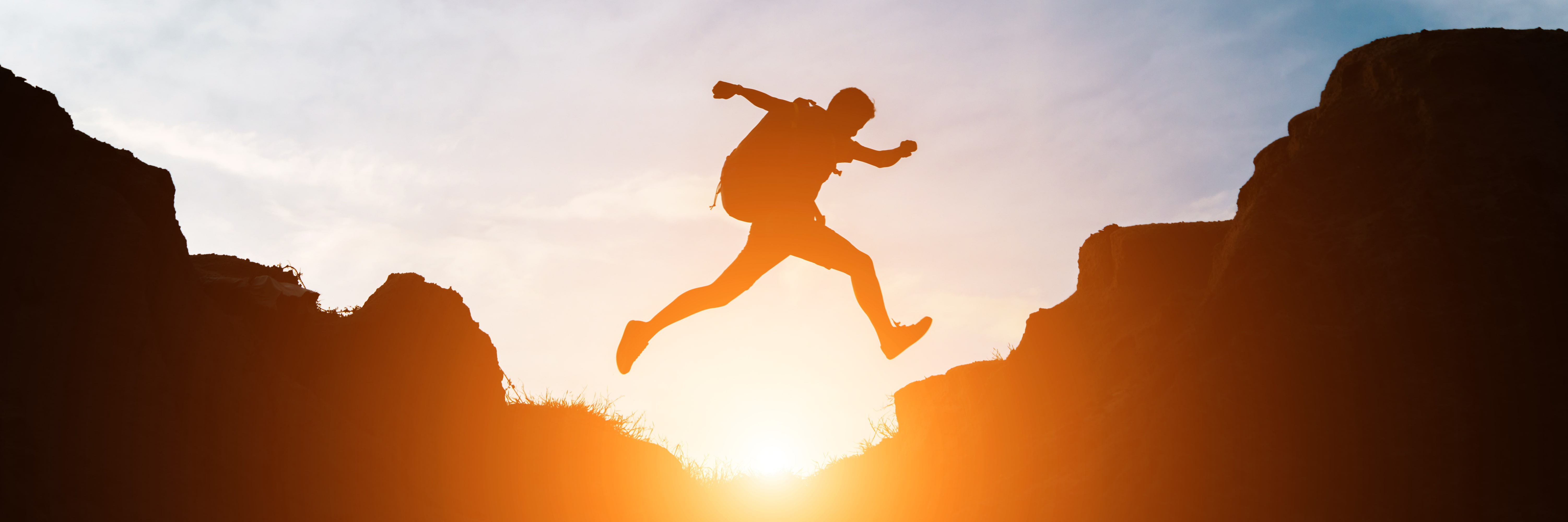
Custom Fullscreen Button: A Cross-Browser Solution
Frontend
Frontend
Fullscreen functionality is a common requirement in web development, especially with media elements like custom video player. In this blog post, I'll share my experience working with fullscreen API
TL;DR
Note: On iOS browsers, element must be a video element . On other browsers, it can be a div/video/... element.
TL;DR
Note: On iOS browsers, element must be a video element . On other browsers, it can be a div/video/... element.
// language: javascript export const openFullScreen = (element) => { if (element.requestFullscreen) { element.requestFullscreen(); } else if (element.mozRequestFullScreen) { /* Firefox */ element.mozRequestFullScreen(); } else if (element.webkitEnterFullScreen) { /* iOS browsers */ element.webkitEnterFullScreen(); } else if (element.webkitRequestFullscreen) { /* Safari/Chrome/Edge */ element.webkitRequestFullscreen(); } else if (element.msRequestFullscreen) { /* IE11 */ element.msRequestFullscreen(); } };
// language: javascript export const closeFullScreen = () => { if (document.exitFullscreen) { document.exitFullscreen(); } else if (document.mozCancelFullScreen) { /* Firefox */ document.mozCancelFullScreen(); } else if (document.webkitExitFullscreen) { /* Safari/Chrome/Edge */ document.webkitExitFullscreen(); } else if (document.msExitFullscreen) { /* IE11 */ document.msExitFullscreen(); } };
Explain and notes
Each browser has its fullscreen API, so you must include all of them to ensure it works on different browsers.
However, iOS browsers, including Safari and Chrome, have a limitation: they only allow video element to go fullscreen. If you try to use the standard Fullscreen API on a div element, it won't work.
You can use package react-device-detect to detect iOS browsers
However, iOS browsers, including Safari and Chrome, have a limitation: they only allow video element to go fullscreen. If you try to use the standard Fullscreen API on a div element, it won't work.
You can use package react-device-detect to detect iOS browsers
// language: javascript import { isIOS } from 'react-device-detect' const handleFullScreenClick = () => { const fullscreenElement = isIOS ? videoElement : containerElement isFullScreen ? closeFullScreen() : openFullScreen(fullscreenElement) }
How can we get isFullScreen status? Should we update it to true/false when the user clicks the custom fullscreen button?
The answer is no.
Users can exit fullscreen mode by hitting the ESC button without clicking the fullscreen button. So you should update isFullScreen status by listening to the below event:
// language: javascript document.addEventListener('fullscreenchange', () => { const isInFullScreen = !!document.fullscreenElement setIsFullScreen(isInFullScreen) })
Conclusion
Because fullscreen API depends on browser type, please test your fullscreen button on different browsers carefully before production release.
I use BrowserStack to test browser compatibility, you can give it a try.
Ref: Fullscreen API - Web APIs | MDN (mozilla.org)
I use BrowserStack to test browser compatibility, you can give it a try.
Ref: Fullscreen API - Web APIs | MDN (mozilla.org)
Created at
2024-01-27 19:06:20 +0700
Related blogs

[PART 1] Unlocking React Interview: Key Questions and In-Depth Answers
React.js is one of the most popular JavaScript libraries for building user interfaces. Whether you're a seasoned developer or just starting out, under...
Frontend
Frontend
2024-06-17 21:16:52 +0700

[PART 1] Unlocking JavaScript Interview: Promises and Timers
JavaScript interviews often include questions about asynchronous programming, as it's a fundamental concept in modern web development. One common chal...
Frontend
Frontend
2024-06-23 16:51:19 +0700